How to Add CSS Link to HTML
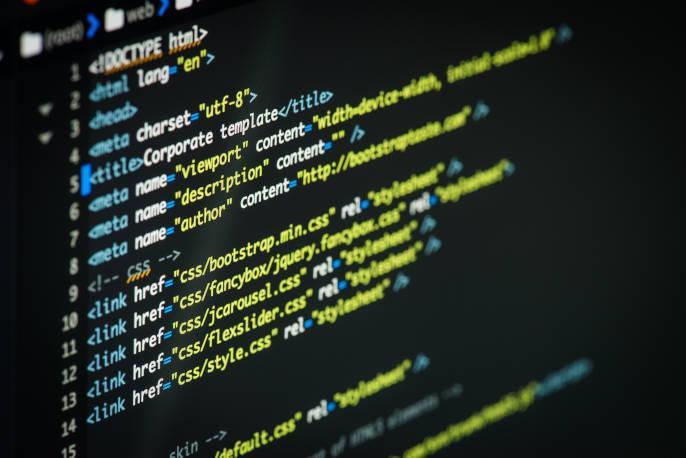
Adding CSS links to HTML
Published on: (Updated on: )
CSS is the magic that beautifies web pages. Here, we'll cover adding CSS to HTML with code examples. CSS is vital for colors, layouts, and more. But first, connect your HTML to CSS.
The <link>
Element in HTML
To link an external CSS file, use the <link> element in the <head> section.. This is often placed within the <head>
section of your HTML page. Here's the basic syntax:
<link rel="stylesheet" type="text/css" href="path-to-your-css-file.css">
- "rel" attribute set to "stylesheet" connects HTML and the stylesheet.
- "type" attribute set to "text/css" specifies the file's type.
- "href" attribute points to the CSS file's location.
Below are sample codes showing how to add CSS link to HTML
Let's assume you have an HTML file named index.html
and a CSS file named styles.css
in the same directory.
Here’s how you would link them together:
index.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Sample Web Page</title>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
<body>
<h1>Welcome to My Web Page</h1>
<p>This is a sample paragraph.</p>
</body>
</html>
styles.css
:
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
}
h1 {
color: #333;
}
p {
color: #666;
}
In this example, the styles.css
file will give the webpage a specific font family, a light gray background, and specific colors for the header and paragraph. The link in the index.html
file ensures that these styles are applied when the webpage is viewed.
Other Methods for adding CSS to an HTML file
While linking to an external CSS file is the most common method, there are two other ways to include CSS in your HTML:
- Internal (or Embedded) CSS: You can include CSS directly in your HTML document using the
<style>
tag within the<head>
section. This is useful when styling a single document without needing an external file.
<style>
body {
background-color: lightblue;
}
</style>
Inline CSS: This involves adding styles directly to individual HTML elements using the style
attribute. It's useful for single-element styling but isn't recommended for larger projects as it can become messy.
<p style="color:red;">This is a red paragraph.</p>
Conclusion
Mastering CSS and HTML linking is fundamental in web development. It separates content (HTML) from presentation (CSS), leading to cleaner, manageable code. Whether it's a small project or a large website, this skill brings your designs to life.