How to Implement Debouncing in Next.js
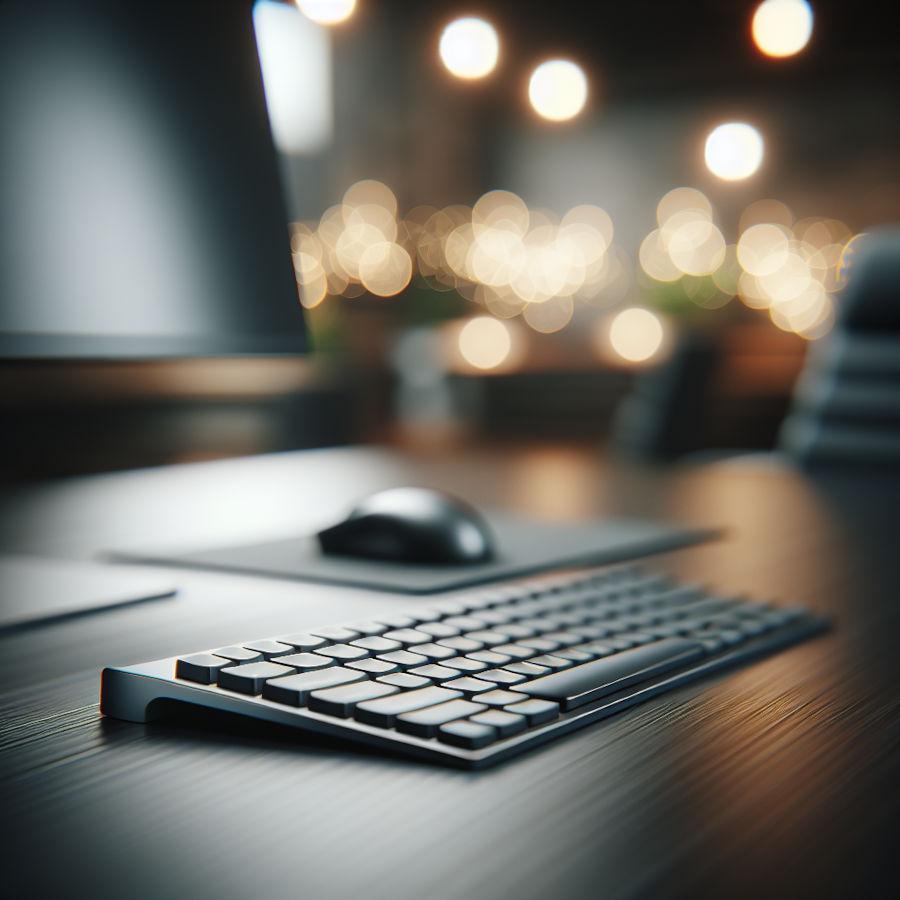
Published on: (Updated on: )
In today’s fast-paced web environment, creating a seamless user experience is vital. One common challenge developers face is handling repetitive user actions that can lead to performance issues or data inaccuracies. This is where debouncing becomes a powerful technique.
What is Debouncing?
Debouncing is a programming pattern that ensures a function is not called too frequently. It delays the execution of a function until after a specified period of time has passed since it was last invoked. This technique helps to limit the number of times a function is executed, especially when it is triggered by popular events like rapid button clicks or repeated API requests.
Why Use Debouncing?
By implementing debouncing, you can:
- Prevent Duplicate Actions: Avoid multiple submissions or requests caused by user impatience (like double-clicking).
- Enhance Performance: Reduce the number of requests made to the server, optimizing bandwidth and server resources.
- Improve User Experience: Provide smoother interactions by preventing visual clutter and unnecessary server interactions.
Real-World Example: Implementing Page View Counts
Let’s take a look at a common requirement in web applications: tracking page view counts. When users navigate through pages, you may want to track how many times a page is viewed. Without debouncing, each navigation might trigger multiple tracking requests, which can lead to inaccurate counts and overwhelming your server.
Step-by-Step Implementation
1. Setting Up Your Environment
First, ensure you have a modern JavaScript/TypeScript environment. For this example, we will use a Next.js application.
npx create-next-app@latest my-app
cd my-app
2. Create the Page View Count API Endpoint
Create an API endpoint to handle page view count requests. This endpoint will manage the logic for incrementing the view counts.
// pages/api/pageview.ts
import { NextRequest, NextResponse } from 'next/server';
// Simulated in-memory store for page views
let pageViewCounts: Record<string, number> = {};
export async function POST(request: NextRequest) {
const { page } = await request.json();
if (!page) {
return NextResponse.json({ error: "No page specified" }, { status: 400 });
}
// Increment the page view count
pageViewCounts[page] = (pageViewCounts[page] || 0) + 1;
return NextResponse.json({ success: `${page} view count updated to ${pageViewCounts[page]}` });
}
3. Implement the Debounce Logic
Now, let’s implement debouncing in a component that will track page views.
// components/PageViewTracker.tsx
import React, { useEffect } from 'react';
import { API_BASE } from '@/services/endpoints';
let lastRequestTime: number | null = null;
const PageViewTracker = ({ page }: { page: string }) => {
const debounceDelay = 2000; // 2 seconds
// Debounce function to track page views
const trackPageView = async () => {
const currentTime = Date.now();
// Debounce logic
if (lastRequestTime && (currentTime - lastRequestTime < debounceDelay)) {
console.log("Duplicate page view request ignored due to debouncing.");
return;
}
lastRequestTime = currentTime; // Update the last request time
try {
const response = await fetch(`${API_BASE}/api/pageview`, {
method: 'POST',
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json',
},
body: JSON.stringify({ page }),
});
if (!response.ok) {
console.error("Error tracking page view");
}
} catch (error) {
console.error("Failed to track page view:", error);
}
};
// Track page views when component mounts or page changes
useEffect(() => {
trackPageView();
}, [page]);
return null; // This component doesn't render anything visible
};
export default PageViewTracker;
4. Include the Page View Tracker in Your Pages
Integrate the PageViewTracker component in your pages to start tracking when they are viewed.
// pages/index.tsx (or any other page)
import PageViewTracker from '../components/PageViewTracker';
export default function Home() {
return (
<div>
<h1>Welcome to My App</h1>
<PageViewTracker page="home" />
{/* Other content goes here */}
</div>
);
}
Conclusion
Debouncing is a vital technique for managing user interactions in web applications. In our page view count example, implementing debouncing prevents multiple tracking requests from being sent when users navigate quickly or refresh the page.